#include <Adafruit_CC3000.h>
#include <ccspi.h>
#include <SPI.h>
#include <string.h>
#include "utility/debug.h"
#include "Adafruit_WS2801.h"
// These are the interrupt and control pins
#define ADAFRUIT_CC3000_IRQ 3 // MUST be an interrupt pin!
// These can be any two pins
#define ADAFRUIT_CC3000_VBAT 5
#define ADAFRUIT_CC3000_CS 10
// Use hardware SPI for the remaining pins
// On an UNO, SCK = 13, MISO = 12, and MOSI = 11
Adafruit_CC3000 cc3000 = Adafruit_CC3000(ADAFRUIT_CC3000_CS, ADAFRUIT_CC3000_IRQ, ADAFRUIT_CC3000_VBAT,
SPI_CLOCK_DIVIDER); // you can change this clock speed
#define WLAN_SSID "chae" // cannot be longer than 32 characters!
#define WLAN_PASS "0312972799"
// Security can be WLAN_SEC_UNSEC, WLAN_SEC_WEP, WLAN_SEC_WPA or WLAN_SEC_WPA2
#define WLAN_SECURITY WLAN_SEC_WPA2
#define IDLE_TIMEOUT_MS 3000 // Amount of time to wait (in milliseconds) with no data
// received before closing the connection. If you know the server
// you're accessing is quick to respond, you can reduce this value.
// What page to grab!
#define WEBSITE "api.openweathermap.org"
#define WEBPAGE "/data/2.5/weather?q=anyang&mode=xml&APPID=1632acd3a9f8d66d1be9ea8b7fd8dc9f"
/**************************************************************************/
/*!
@brief Sets up the HW and the CC3000 module (called automatically
on startup)
*/
/**************************************************************************/
Adafruit_CC3000_Server server(80);
Adafruit_CC3000_Client www = cc3000.connectTCP(ip, 80);
if (www.connected()) {
www.fastrprint(F("GET "));
www.fastrprint(WEBPAGE);
www.fastrprint(F(" HTTP/1.1\r\n"));
www.fastrprint(F("Host: ")); www.fastrprint(WEBSITE); www.fastrprint(F("\r\n"));
www.fastrprint(F("\r\n"));
www.println();
} else {
Serial.println(F("Connection failed"));
return;
}
Serial.println(F("-------------------------------------"));
unsigned long lastRead = millis();
while (www.connected() && (millis() - lastRead < IDLE_TIMEOUT_MS)) {
while (www.available()) {
char c = www.read();
Serial.print(c);
lastRead = millis();
}
}
www.close();
Serial.println(F("-------------------------------------"));
/* You need to make sure to clean up after yourself or the CC3000 can freak out */
/* the next time your try to connect ... */
Serial.println(F("\n\nDisconnecting"));
cc3000.disconnect();
IPAddress hostIp;
uint32_t ip;
unsigned long lastAttemptTime = 0; // 마지막으로 서버에서 데이터를 전송받은 시간
String currentLine = "";
String weatherString = "";
String timeString = "";
String location = "kiev";
boolean readingWeather = false;
boolean readingTime = false;
boolean stringComplete = false;
int weather;
int temp = 0;
uint8_t dataPin = 2; // Yellow wire on Adafruit Pixels
uint8_t clockPin = 3; // Green wire on Adafruit Pixels
int lednum = 30;
Adafruit_WS2801 strip = Adafruit_WS2801(25, dataPin, clockPin);
void setup(void)
{
Serial.begin(115200);
#if defined(__AVR_ATtiny85__) && (F_CPU == 16000000L)
clock_prescale_set(clock_div_1); //clock을 16Mhz로 사용
#endif
strip.begin();
strip.show();
delay(10);
Serial.println(F("Hello, CC3000!\n"));
Serial.print("Free RAM: "); Serial.println(getFreeRam(), DEC);
/* Initialise the module */
Serial.println(F("\nInitializing..."));
if (!cc3000.begin())
{
Serial.println(F("Couldn't begin()! Check your wiring?"));
while(1);
}
// Optional SSID scan
// listSSIDResults();
Serial.print(F("\nAttempting to connect to ")); Serial.println(WLAN_SSID);
if (!cc3000.connectToAP(WLAN_SSID, WLAN_PASS, WLAN_SECURITY)) {
Serial.println(F("Failed!"));
while(1);
}
Serial.println(F("Connected!"));
/* Wait for DHCP to complete */
Serial.println(F("Request DHCP"));
while (!cc3000.checkDHCP())
{
delay(100); // ToDo: Insert a DHCP timeout!
}
/* Display the IP address DNS, Gateway, etc. */
while (! displayConnectionDetails()) {
delay(1000);
}
ip = 0;
// Try looking up the website's IP address
Serial.print(WEBSITE); Serial.print(F(" -> "));
while (ip == 0) {
if (! cc3000.getHostByName(WEBSITE, &ip)) {
Serial.println(F("Couldn't resolve!"));
}
delay(500);
}
cc3000.printIPdotsRev(ip);
// Optional: Do a ping test on the website
/*
Serial.print(F("\n\rPinging ")); cc3000.printIPdotsRev(ip); Serial.print("...");
replies = cc3000.ping(ip, 5);
Serial.print(replies); Serial.println(F(" replies"));
*/
/* Try connecting to the website.
Note: HTTP/1.1 protocol is used to keep the server from closing the connection before all data is read.
*/
Adafruit_CC3000_Client www = cc3000.connectTCP(ip, 80);
if (www.connected()) {
www.fastrprint(F("GET "));
www.fastrprint(WEBPAGE);
www.fastrprint(F(" HTTP/1.1\r\n"));
www.fastrprint(F("Host: ")); www.fastrprint(WEBSITE); www.fastrprint(F("\r\n"));
www.fastrprint(F("\r\n"));
www.println();
} else {
Serial.println(F("Connection failed"));
return;
}
Serial.println(F("-------------------------------------"));
/* Read data until either the connection is closed, or the idle timeout is reached. */
}
void loop(void)
{
if (client.connected()) {
while (client.available()) {
//전송된 데이터가 있을 경우 데이터를 읽어들인다.
char inChar = client.read();
// 읽어온 데이터를 inChar에 저장한다.
currentLine += inChar;
//inChar에 저장된 Char변수는 currentLine이라는 String변수에 쌓이게 된다.
//라인피드(줄바꿈)문자열이 전송되면 데이터를 보내지 않는다.
if (inChar == '\n') {
//Serial.print("clientReadLine = ");
//Serial.println(currentLine);
currentLine = "";
}
//온도 데이터가 전송되었는지 확인
if ( currentLine.endsWith("<temperature value=")) {
//현재 스트링이 "<temperature value="로 끝났다면 온도데이터를 받을 준비를 한다.
readingTemp = true;
tempString = "";
}
//<temperature value=뒤에 오는 문자열을 tempString에 저장한다.
if (readingTemp) {
if (inChar != 'm') { //전송될 문자가 'm'이 올때까지 온도값으로 인식
tempString += inChar;
}
else { //전송된 문자가 'm'이라면 온도데이터를 그만 저장하고 온도값 출력
readingTemp = false;
Serial.print("- Temperature: ");
Serial.print(getInt(tempString)-273);
Serial.println((char)176); //degree symbol
}
}
if ( currentLine.endsWith("<humidity value=")) {
//현재 스트링이 "<humidity value ="로 끝났다면 습도 데이터를 받을 준비를 한다.
readingHum = true;
humString = "";
}
if (readingHum) {
if (inChar != 'u') {//전송될 문자열이 'u'가 아니라면 계속 습도값을 받게 된다.
humString += inChar;
}
else { //다음에 전송된 문자열이 'u'라면 습도값을 그만 받고 값을 출력한다.
readingHum = false;
Serial.print("- Humidity: ");
Serial.print(getInt(humString));
Serial.println((char)37);
}
}
if ( currentLine.endsWith("<lastupdate value=")) {
// 현재 스트링이 "<lastupdata value="로 끝났다면 마지막 업데이트 시간 데이터를 받을 준비를 한다.
readingTime = true;
timeString = "";
}
if (readingTime) {
if (inChar != '/') { //다음 전송될 문자가 '/'가 아니라면 계속적으로 시간데이터를 받는다
timeString += inChar;
}
else {
readingTime = false;
Serial.print("- Last update: ");
Serial.println(timeString.substring(2,timeString.length()-1));
}
}
if ( currentLine.endsWith("<pressure value=")) {
// 현재 스트링이 "<pressure value="로 끝났다면 기압 데이터를 받을 준비를 한다.
readingPressure = true;
pressureString = "";
}
if (readingPressure) {
if (inChar != 'u') { //다음 전송될 문자가 'u'가 아니라면 계속 기압데이터를 받는다.
pressureString += inChar;
}
else { //다음 전송된 문자가 'u'라면 기압데이터를 출력한다.
readingPressure = false;
Serial.print("- Pressure: ");
Serial.print(getInt(pressureString));
Serial.println("hPa");
}
}
if ( currentLine.endsWith("</current>")) { //현재 스트링이 </current>로 끝났다면 연결을 끊고 다시 서버와 연결을 준비한다.
delay(10000); //10초뒤에 서버와 연결을 끊고 재연결을 시도한다.
client.stop();
connectToServer();
//Serial.println("Disconnected from Server.\n");
}
}
}
else if (millis() - lastAttemptTime > requestInterval) {
//연결을 실패했다면 requestInterval(60초)이후에 다시 연결을 시도한다.
connectToServer();
}
}
/**************************************************************************/
/*!
@brief Begins an SSID scan and prints out all the visible networks
*/
/**************************************************************************/
void listSSIDResults(void)
{
uint32_t index;
uint8_t valid, rssi, sec;
char ssidname[33];
if (!cc3000.startSSIDscan(&index)) {
Serial.println(F("SSID scan failed!"));
return;
}
Serial.print(F("Networks found: ")); Serial.println(index);
Serial.println(F("================================================"));
while (index) {
index--;
valid = cc3000.getNextSSID(&rssi, &sec, ssidname);
Serial.print(F("SSID Name : ")); Serial.print(ssidname);
Serial.println();
Serial.print(F("RSSI : "));
Serial.println(rssi);
Serial.print(F("Security Mode: "));
Serial.println(sec);
Serial.println();
}
Serial.println(F("================================================"));
cc3000.stopSSIDscan();
}
/**************************************************************************/
/*!
@brief Tries to read the IP address and other connection details
*/
/**************************************************************************/
bool displayConnectionDetails(void)
{
uint32_t ipAddress, netmask, gateway, dhcpserv, dnsserv;
if(!cc3000.getIPAddress(&ipAddress, &netmask, &gateway, &dhcpserv, &dnsserv))
{
Serial.println(F("Unable to retrieve the IP Address!\r\n"));
return false;
}
else
{
Serial.print(F("\nIP Addr: ")); cc3000.printIPdotsRev(ipAddress);
Serial.print(F("\nNetmask: ")); cc3000.printIPdotsRev(netmask);
Serial.print(F("\nGateway: ")); cc3000.printIPdotsRev(gateway);
Serial.print(F("\nDHCPsrv: ")); cc3000.printIPdotsRev(dhcpserv);
Serial.print(F("\nDNSserv: ")); cc3000.printIPdotsRev(dnsserv);
Serial.println();
return true;
}
}
말씀하신대로 Adafruit_CC3000_Client www = cc3000.connectTCP(ip, 80); 클라이언트부분으로 설정하였는데
다음 에러가 나오네요 봐주세요 ㅜ
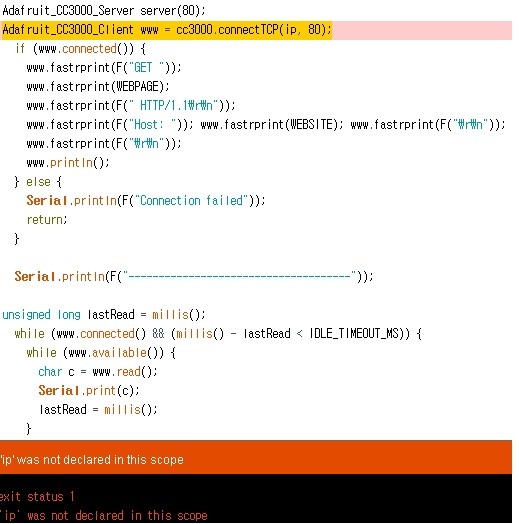
|